Dependency Injection and Policy Based Design
I believe that one indicator that an approach or technique is a good idea is that it appears in multiple contexts or gets invented simultaneously by different people. The dependency injection pattern is an example of this because of its similarity to policy-based design. This helps reinforce my feelings that both approaches are worthwhile techniques for reducing code coupling. </p>
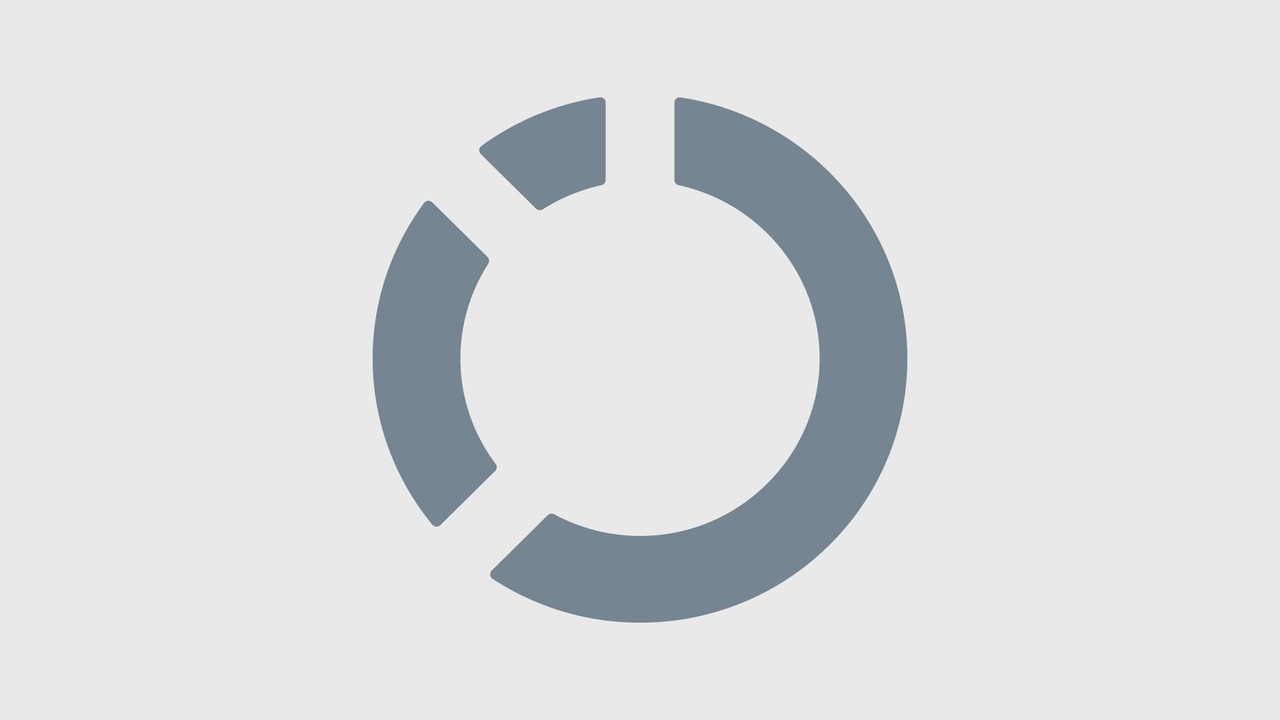
I believe that one indicator that an approach or technique is a good idea is that it appears in multiple contexts or gets invented simultaneously by different people. The dependency injection pattern is an example of this because of its similarity to policy-based design. This helps reinforce my feelings that both approaches are worthwhile techniques for reducing code coupling.
I recently came upon this post (http://skepticalmethodologist.wordpress.com/dependency-injection-in-c/) describing the dependency injection pattern in C++ as ported from Java. The dependency injection pattern uses abstract classes and factories rather than concrete classes within a class to reduce coupling and improve the flexibility of a design.
Here is an example of where we might want to use the dependency injection pattern. First is the straightforward approach to implementing a class that uses another concrete class as a field.
class MyOtherClass {
int m;
public:
MyOtherClass(int x) : m(x) { }
int DoSomething() { return m + 1; }
};class MyClass {
MyOtherClass m;
public:
MyClass() : m(42) { }
int DoSomething() { return m.DoSomething(); }
};
Now here is an dependency injection pattern ported naively from Java:
class AbstractMyOtherClass {
public:
virtual int DoSomething() = 0;
virtual ~AbstractMyOtherClass() { delete(m); }
};class MyOtherClass : AbstractMyOtherClass {
int m;
public:
MyOtherClass(int x) : m(x) { }
int DoSomething() { return m + 1; }
};class MyOtherClassFactory {
AbstractMyOtherClass* Create(int x) {
return new MyOtherClass(x);
}
};
struct MyClass {
boost::scoped_ptr<AbstractMyOtherClass> m;
public:
MyClass(MyOtherClassFactory x) : m(x.Create(42)) { }
int DoSomething() { return m->DoSomething(); }
}
So why not a boost::shared_ptr you might ask? Well you should use it only if you must. Ownership in this case is very clearly linked to "MyClass", and this is where it should stay. Using boost::shared_ptr would be adding an undue ambiguity (i.e. when does the object get deleted) to your implementation.
So we now have an example of the dependency injection pattern. There are however some rather large problems with this new design: we needed to add an object factory (MyOtherClassBuilder) and an abstract base class (AbstractMyOtherClass). I don't think that that is acceptable, because it requires too much scaffolding code just to reduce a little bit of coupling. We are passing the point of diminishing returns.
This approach though points naturally to the following improved implementation:
template<typename Field = MyOtherClass, typename Factory = DefaultFactory<Field> >
class MyClass {
public:
boost::scoped_ptr<Field> m;
MyClass() : m(Factory().Create(42)) { }
int DoSomething() { return m->DoSomething(); }
};
This is a very flexible design and doesn't require a substantial rewriting of existing code. I find it interesting that it is also essentially the same approach used in the STL for the standard collections, where they call the factory an allocator. The following simple implementation of DefaultFactory can be used for a large number of cases:
template<typename T>
struct DefaultFactory {
T* Create() { return new T(); }
template<typename U>
T* Create(U x) { return new T(x); }
// ...
// add more versions of Create as needed
};
With C++ 0x we will be able to make an even better DefaultFactory class by resolving the forwarding problem using variadic template parameters.
To make this a more clear example of Policy based design, we would use a single policy template parameter that combines the other effect. For example:
struct DefaultPolicy {
typedef MyOtherClass Field;
typedef DefaultFactory<Field> Factory;
};template<typename Policy = DefaultPolicy>
class MyClass {
public:
boost::scoped_ptr<Field> m;
MyClass() : m(Factory().Create(42)) { }
int DoSomething() { return m.DoSomething(); }
};
So that's my approach to performing dependency injection using policy-based design. This is a bit of an oversimplification and surely contains several errors (my C++ is really rusty). I would be interested in hearing suggestion on different ways we can improve the code or design, and still achieve a benefit of reducing coupling between classes.
About the Author(s)
You May Also Like