Dr. Dobb's Report: Real-Time Ready Java
Predictable, real-time performance is possible By Eric J. Bruno
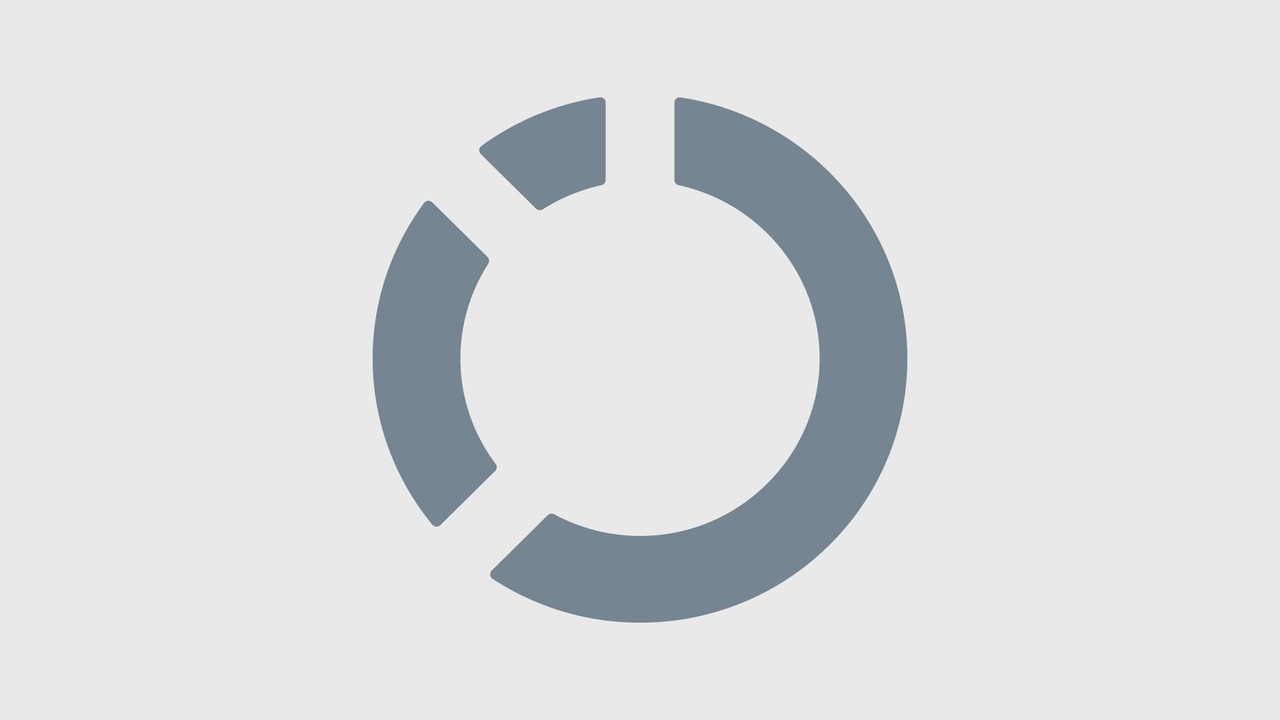
Java has long been one of the central technologies of enterprise applications. The speed and scalability of the JVM, in particular, have endeared it to large IT organizations. But today, companies need more than just fast performance; they are increasingly searching for deterministic, real-time performance.
Determinism in this sense means that a given action will occur within a fixed time interval, such as delivery of a stock quotation within some number of microseconds. Historically, Java hasn't been used to fill that role, because of some early design decisions in the platform. However, new options and new technologies are enabling IT organizations to use Java for both standard business needs and situations where deterministic, real-time requirements must be met.
Few things generate more confusion in the programming world than discussions of real-time software. Many confuse it with high-performance computing, while others use it to describe any system that pushes data to the user without a user request. These characterizations alone simply aren't accurate. While it's true that both these aspects may be part of real-time system behavior, the definition of a real-time system centers on one word: time. Correctness means producing the right answer, and doing so at a precise moment in time.
Another source of confusion is the difference between hard and soft real-time systems. A hard real-time requirement is one in which a task needs to be completed at or before a certain time, every time it's required, regardless of other factors. A soft real-time system contains some tolerance in terms of missing its deadline.
For example, stating that a foreign exchange trade needs to settle within two days is a hard real-time requirement, whereas stating that video player software needs to update its frame 60 times per second is a soft real-time requirement--occasionally dropping a frame isn't considered an error. However, it's still a real-time system because too many dropped frames, or too much delay between them, is considered an error.
Java And Real-Time Development
Java Standard Edition (Java SE) is not ideally suited for real-time requirements. Existing Java Virtual Machines (JVMs) just aren't designed for predictability and determinism.
For instance, garbage collection (GC), in which the RAM allocated to no-longer-used data items is reclaimed for reuse by the JVM, is one source of trouble. GC can occur at any time, for any length of time, with few options for users to control it. This potential for unbounded delays makes it impossible to guarantee a system's behavior; it is nondeterministic. Attempts to pool objects or somehow control their lifetime to avoid GC are often in vain, as GC pauses may still occur.
However, Java SE's real-time deficiencies go beyond the garbage collector. The just-in-time (JIT) HotSpot compiler--the same one that compiles bytecode to machine code--is also nondeterministic. Because JIT compilation can occur when your code is executing, and can take an unknown length of time to complete, you can't be guaranteed that your code will meet its deadlines all the time. Even Java classes that have been JIT-compiled are subject to reoptimization at any point in the future.
More importantly, Java provides no guaranteed way to prioritize threads or event handling within your application code. Therefore, even if GC and JIT could be controlled, real-time behavior couldn't be guaranteed without the ability to prioritize your thread processing. With strict priority control comes the need for advanced locking beyond what most standard JVMs provide. These are important points to remember as most people blame the GC and JIT compiler entirely for Java's lack of real-time ability.
The C++ Alternative
A common alternative to Java for real-time development is C++, but this is a flawed solution. While C++ is a good language, it isn't an entity that magically yields predictability and determinism. It requires great skill and enormous knowledge, along with operating system support and integration, to deliver a real-time system in C++.
For instance, although GC problems don't exist in C++, the C runtime on which C++ depends for heap management can exhibit nondeterministic behavior. Quick examination of some standard C runtime library code reveals that locating free memory chunks for use by the program can involve extensive memory manipulation. This can lead to unpredictable results during memory operations.
Instead, with C++, the developer bears a great burden to ensure deterministic, predictable execution across all aspects of application processing. This usually results in a dependence upon third-party libraries for memory management, thread processing, and interaction with the operating system for I/O operations. These are all features that the Java VM provides built-in. Used correctly, the JVM can perform these operations while providing support for real-time development. Here's how.
Real-Time Java Solutions
In the late '90s, a group of real-time and programming language experts from around the globe worked together to define a specification to define how Java should behave in the real-time space. The result was the Real-Time Specification for Java (RTSJ), which doesn't change the Java language at all, but instead outlines areas of enhancement to the platform to meet real-time requirements. These include:
>> Thread scheduling: The RTSJ states that a real-time scheduler be used to schedule tasks, but it doesn't specify the algorithm, nor how to do it. RTSJ implementations typically rely upon and work with the OS to achieve this goal. RTSJ does, however, define new thread types--i.e. RealtimeThread (RTT), and NoHeapRealtimeThread (NHRT)--for real-time Java execution.
>> Memory management: The RTSJ doesn't require a garbage collector, nor does it specify any algorithms for it. Instead, it defines new memory regions beyond the heap, and specifies that the collector not interfere with them. Therefore, it's possible to perform memory management outside the scope of the Java GC.
>>Resource sharing: With enhanced thread scheduling comes the need for thread priority control. The RTSJ requires the priority inheritance protocol be implemented through the Java synchronized keyword, along with a set of wait-free queues.
>> Asynchronous execution control: To control asynchronous event handling, the RTSJ defines how event-handling code is to be scheduled and dispatched deterministically. It also extends the Java Exception handler to allow immediate shifts of execution within a real-time thread. Finally, it defines a way to terminate a thread's execution safely and deterministically.
>> Physical memory access: The ability to create and access objects within specific regions of physical memory is defined, allowing Java code to interact with I/O and other hardware devices deterministically, and with minimal latency.
The overarching goal of the RTSJ is not to change the Java language (for example, it included no new keywords) but to allow the average Java developer to build real-time software. However, that's not to say some changes in coding practice aren't required. Let's take a quick look at how the RTSJ affects Java programming.
RTSJ In Practice
The Java developer needs to embrace some new concepts to use RTSJ. For instance, the two new thread classes described previously require developers to specify certain aspects of their behavior. These include priority, scheduling behavior (periodic or aperiodic), and memory region requirements. For instance, while an RTT can access objects that reside anywhere, an NHRT can't access objects that reside on the heap.
The RTSJ defines additional memory regions where objects can reside, to ensure no interference with the Java garbage collector. These regions include ScopedMemory, ImmortalMemory, and PhysicalMemory. A ScopedMemory region is an area of memory outside of the Java heap that can be defined and created at runtime, and within which Java objects are created. When the real-time code finishes with the region (something the developer controls), the entire region and the objects it contains are marked as free, and all references to them are removed; no GC is required.
The sole ImmortalMemory region is an area of memory where Java objects live for the life of the VM. Objects created here are never collected, nor are they freed in any way. Hence, ImmortalMemory is a limited resource meant to provide deterministic access to data commonly needed within a real-time Java application.
There are sometimes complex rules for object reference and access between the various memory regions, but I won't go into those details here. Those details as well as asynchronous event handling, asynchronous transfer of control, and physical memory access can be reviewed in the RTSJ document or in one of the books written on the topic.
Real-Time Java Implementations
RTSJ has seen a few revisions over the years, and it's still actively being improved. For instance, JSR-282 is in the early draft review stage, and will define version 1.1 of the RTSJ. Be sure to look at the current specification, and then the proposed revision, to get a feel for where things are headed.
Officially compliant RTSJ implementations include the Sun (now Oracle) Java Real-Time System, the IBM WebSphere Real-Time VM, and the Timesys RTSJ reference implementation. Both Oracle and IBM provide support for multiple operating systems, including Solaris and specific Linux distributions (real-time scheduler required).
There are no RTSJ-compliant implementations available for Windows, but not because of the common misconception that Windows can't provide real-time behavior. To some degree it can, via its real-time thread support, but it simply doesn't provide enough real-time thread priority levels to meet the RTSJ's requirements.
The common Linux distributions also don't meet the RTSJ's requirements for real-time systems. Instead, IBM provides its own real-time Linux variant to guarantee real-time behavior, and Oracle requires you use either Red Hat's Messaging-Realtime-Grid Linux distribution, or Novell's Suse Linux Enterprise Linux extensions for Suse Linux Enterprise Server.
Real-Time, Without RTSJ
There are other real-time Java implementations that aren't strictly RTSJ-compliant, although they may implement most or part of the API. These include:
>> Aicas JamaicaVM: A Java SE/ RTSJ-compatible implementation with a real-time, deterministic garbage collector.
>> Aonix PERC: A Java SE/RTSJ-compatible implementation with its own real-time, deterministic garbage collector. Aonix also has support for embedded devices with memory limitations, as well as systems with safety-critical requirements.
>> Fiji: A small-footprint Java implementation for embedded systems with deterministic garbage collection and support for safety-critical systems.
>> Javolution: A real-time Java library that provides a set of classes for deterministic execution and RTSJ support.
Again, true real-time Java development goes beyond the need for just real-time garbage collection. When choosing a real-time Java VM, whether it's RTSJ-compliant or not, be sure to choose one that guarantees your application will meet time-based requirements, with enough support to deterministically schedule your application's processing. Let's take a look at some areas real-time Java is being used today.
Business Success Cases
I've evaluated and deployed real-time Java in a wide range of applications. These include financial applications, such as trading engines, quote publishing, and news delivery; military systems, such as object tracking and flight control; telecommunication systems; and other specialized projects, including embedded systems, robotics, and embedded controllers.
Specific projects include a trading system developed at Reuters, embedded systems development at Perrone Robotics, a system for tracking space objects at ITT, flight systems at Boeing, and various military projects.
Most of these projects have been a big improvement over using specialized languages and programming environments that would have otherwise been required. Projects where real-time Java sometimes doesn't fit are ones where non-real-time requirements are mixed in, such as the need for high transaction rates and overall throughput. These are two areas that often require a trade-off to achieve predictable real-time behavior.
As tight time requirements become a greater part of enterprise computing, it's helpful to know that the same Java language and tooling used for developing standard applications can also be used to create deterministic, real-time computing solutions.
Eric J. Bruno is the author of "Real-Time Java Programming" and a blogger for Dr. Dobb's Journal. Write to us at [email protected].
About the Author(s)
You May Also Like